Hi-Lo- Hangman Game
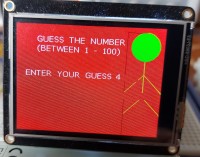
This is a Hi-Lo Hangman game played using the MAX78000FTHR development board. A secret number is generated by the processor. You are required to guess this number by giving the digits of your numbers as voice commands. The processor displays hints to help you guess the number. A hangman figure is built part by part as you give wrong guesses. You have 10 attempts before being hanged!.
Video of the project at link: https://www.youtube.com/watch?v=th3j-VCM5QE
Hi-Lo Hangman Game: This project is based on the concepts of artificial intelligence where a voice activated game is developed using the MAX78000FTHR microcontroller development board. The program generates an integer secret random number between 1 and 100. The user is expected to guess this number by entering numbers by voice. Every time an answer is wrong part of a hangman figure is built. The user has 10 attempts to guess the secret number. After 10 failed attempts the full hangman figure will be built and the hangman will be hanged and this will be the end of the game where a new secret number will be generated and the game will continue.
The program displays hints to the user as follows:
TRY MUCH HIGHER: the number you guessed is very low. Try a much higher number (difference between the secret number and your guess is equal to or greater than 50)
TRY HIGHER: the number you guessed is low. Try a higher number (difference between the secret number and your guess is equal to or greater than 20)
TRY SLIGHTLY HIGHER: the number you guessed is slightly low. Try a slightly higher number (difference between the secret number and your guess is equal to or greater than 5)
VERY CLOSE!: the number you guessed is very close to the secret number (difference between the secret number and your guess is greater than or equal to 1)
TRY MUCH LOWER: the number you guessed is very high. Try a much lower number (difference between your guess and the secret number is equal to or greater than 50)
TRY LOWER: the number you guessed is high. Try a lower number (difference between your guess and the secret number is equal to or greater than 20)
TRY SLIGHTLY LOWER: the number you guessed is slightly high. Try a slightly lower number (difference between the secret number and your guess is equal to or greater than 5)
VERY CLOSE!: the number you guessed is very close to the secret number (difference between your guess and the secret number is greater than or equal to 1)
At the heart of the MAX78000FTHR is a MAX78000 Cortex-M4 ARM based microcontroller with FPU. Additionally, the board contains 512KB flash memory, 128KB SRAM, 16KB cache, Convolutional Neural Network Accelerator, VGA image sensor, digital microphone, RGB LED, pushbuttons, microSD card adapter, microUSB connector, stereo audio CODEC, SWD debugger, virtual UART port, and many I/O ports.
Block Diagram: Figure 1 shows the block diagram of the project. A 2.4 inch ILI9341 controller based TFT FeatherWing 240x320 pixel touch-screen TFT display is used at the front end of the project to display the user commands and responses.
Circuit Diagram: Figure 2 shows the circuit diagram of the project. The TFT display is connected to the MAX78000FTHR via the SPI bus interface. Pins MISO, MOSI, SCK and CS of the SPI bus are connected to pins P0_6 (MISO), P0_5 (MOSI), P0_7 (SCK), and P0_11 (CS) of the development board. Pin D/C of the TFT display is connected to pin P0_8, and Vcc and GND are connected to +3.3V and GND pins of the MAX78000FTHR.
The circuit was built on a breadboard and connections were made using jumper wires. Figure 3 shows the project built on a breadboard.
Power is supplied to the circuit through its mikroUSB cable which should be connected to the USB port of a computer during the program development.
Operation of the project: The following sounds are recognized by the project (invalid spoken commands are rejected by the project):
Numbers 0 to 9 (entered as voice commands ZERO ONE TWO,…….. NINE)
YES voice command used to terminate the user entry
For example, number 25 is entered by speaking: TWO FIVE YES
You should see the digits displayed on the TFT display as you speak them.
Program listing: The 20 keywords in the supplied Maxim project kws20_demo is used.. The CNN training program was ran on a Linux Ubuntu operating system after creating a WMware virtual machine on a Windows 10 machine with an i7 CPU, 100GB disk space, and 12GB free memory, without a special GeoForce GPU. After training the new words, the created cnn.c, cnn.h files and the weights.c and weights.h files were copied to the workspace and program main.c under folder kws20_demo was modified for this project. The full main.c program listing is given in Figure 4 as a PDF file.
Function Detected_Word detects the spoken words and returns an integer number which is used to identify the detected sound. Number 100 is returned if a non-valid sound is detected by the program. This function body is as follows:
int Detected_Word(int16_t out_class)
{ if(strcmp(keywords[out_class], "YES") == 0) return 10; else if(strcmp(keywords[out_class], "ONE") == 0) return 1; // Numeric sounds..... else if(strcmp(keywords[out_class], "TWO") == 0) return 2; // ... else if(strcmp(keywords[out_class], "THREE") == 0) return 3; // ... else if(strcmp(keywords[out_class], "FOUR") == 0) return 4; // ... else if(strcmp(keywords[out_class], "FIVE") == 0) return 5; // ... else if(strcmp(keywords[out_class], "SIX") == 0) return 6; // ... else if(strcmp(keywords[out_class], "SEVEN") == 0) return 7; // ... else if(strcmp(keywords[out_class], "EIGHT") == 0) return 8; // ... else if(strcmp(keywords[out_class], "NINE") == 0) return 9; // ... else if(strcmp(keywords[out_class], "ZERO") == 0) return 0; // Numeric sounds else
return 100;
}
Random numbers are generated using the MAX78000 processor True Random Number Generator engine (TRNG). The generated number is used as a seed to function srand() so that different number set is generated each time the program is run. Functions rand() are then called to generate the required numbers as shown in the following example:
MXC_TRNG_Random(&rnd8, 1);srand(rnd8);firstno = 1 + rand() % 100; // Generate number between 1 and 100
where the seed is generated outside the main program loop.
The hangman is built by displaying a circle for its head, and by drawing lines for its hands and legs. The colour of the hangman is green. Its head changes to magenta colour when it is hanged!. A switch statement is used to build parts of the hangman every time the user guess is wrong. The following code is used in the switch statement:
hangman++;
switch (hangman
{ case 1: MXC_TFT_FillCircle(280, 40, 30, GREEN);
break;
case 2: MXC_TFT_Line(280, 70, 280, 100, GREEN);
break;
case 3: MXC_TFT_Line(280, 100, 240, 140, GREEN);
break;
case 4: MXC_TFT_Line(280, 100, 320, 140, GREEN);
break;
case 5: MXC_TFT_Line(280, 70, 280, 150, GREEN);
break;
case 6: MXC_TFT_Line(280, 150, 240, 190, GREEN);
break;
case 7: MXC_TFT_Line(280, 150, 320, 190, GREEN);
break;
case 8: MXC_TFT_Line(240, 10, 280, 10, BLACK);
break;
case 9: MXC_TFT_Line(240, 10, 240, 300, BLACK);
break;
case 10: MXC_TFT_FillCircle(280, 40, 30, MAGENTA);
MXC_Delay(SEC(3));
MXC_TFT_ClearScreen();
askflag = 0;
Wrongcount = 0;
Checkno = 0;
Secretno = 0;
break; }
}
Variable Secretno is the secret random number generated by the program and variable Checkno is the number entered (spoken) by the user.
The ELEKTOR lego was converted into a bitmap image and then into a c file, and is displayed when the project is started (see the section on running the project).
Figures 5.1 to 5.11 show snapshots of the TFT display as the game progresses.
Example run of the project: An example run of the project is shown in the You Tube video link. Notice that the ELEKTOR logo is displayed when power is applied to the project, or when the Reset button is pressed. Then, a short screen is displayed as a header which gives very brief information about the project. The user is then prompted to speak the required valid commands.
Video of the project at link: https://www.youtube.com/watch?v=th3j-VCM5QE
Suggestions for future work:
The project can be upgraded by introducing different levels to the project. Also, more tips can be given to the user to make the game more enjoyable.
References:
https://datasheets.maximintegrated.com/en/ds/MAX78000.pdf
https://www.maximintegrated.com/en/design/technical-documents/app-notes/7/7417.html
https://www.maximintegrated.com/en/design/videos.html/vd_1_rtp4xipe#popupmodal
https://datasheets.maximintegrated.com/en/ds/MAX78000FTHR.pdf
https://www.maximintegrated.com/content/dam/files/design/tools/ev-kits/schematics/max78000-fthr-schematic.pdf
Discussie (0 opmerking(en))